How to Convert Decimal Numbers to Binary
In this article, we take a look at what the decimal and binary number systems are, how to convert decimal numbers to binary, and vice versa. We also explain the math behind decimal-binary conversion and list simple programs in Java, JavaScript, and Python for converting decimal to binary numbers.
Table of Contents
- How to convert decimal numbers to binary
- How to convert binary numbers to decimal
- The math behind decimal-binary conversion
- Decimal to binary table
- Programs for converting decimal to binary
Background
The decimal and binary number systems are two of the most used numbers systems across the world. The decimal system is the most familiar to us, and is used almost everywhere in our day-to-day calculations. It has a base of 10 - i.e., 10 numerical characters, or 0, 1, 2, 3, 4, 5, 6, 7, 8 and 9.
On the other hand, the binary number system is used extensively in machine-level programming. It has a base of 2, i.e., two numerical characters - 0 and 1.
Almost every computer and digital device is powered by the binary system, because it is very easy to implement in electronic circuits via logic gates. In computers, every digit of a binary number is called a 'bit'. For example:
- 10 is a 2-bit binary number, where 1 and 0 are bits
- 110 is a 3-bit binary number
- 11011 is a 5-bit binary number
There are several methods and programs for converting decimal numbers to binary, and vice-versa. Let's first take a look at the most common method used for conversion, and then delve deeper into the math behind the decimal-binary conversion.
How to convert decimal numbers to binary
Let's look at two cases - converting decimal integers to binary, and then decimal fractions less than 1 to binary.
Decimal integers to binary: Algorithm
Step 1. Divide the integer by 2, while noting the quotient and remainder.
Step 2. Then, divide the quotient again by 2, and record the 3rd quotient and remainder.
Step 3. Like this, keep dividing each successive quotient by 2 until you get a quotient of zero.
Step 4. After this, just write all the remainders in reverse order to get the binary representation of the integer.
For example, let's convert the integer 15 to binary. Divide the integer by 2 successively while noting the quotient and remainder.
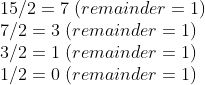
Next, write the all the remainders in reverse order. In this example, remainders are 1, 1, 1, 1. So, writing in reverse order, the number 15 in decimal is represented as 1111 in binary.
For an even integer, let's take the example of 10.
-p4bnr.gif)
So, the decimal number 10 is represented as 1010 in binary.
Decimal fractions to binary: Algorithm
To convert a fraction to binary, follow the opposite of the process described above.
Step 1. Multiply the fraction by 2, while noting down the resulting integer and fraction parts of the product.
Step 2. Keep multiplying each successive resulting fraction by 2 until you get a resulting fraction product of zero.
Step 3. Now, write all the integer parts of the product in each step.
For example, let us convert the fraction 0.625 to binary. Multiply the fraction by 2 successively while noting the integer and fractional parts of the product.
-ld34l.gif)
We see that the resulting integer parts of the product are 1, 0, 1. Hence, just write these after the decimal point to get the binary notation. So, 0.625 can be written as 0.101 in binary.
How to convert binary numbers to decimal
Let's look at two cases - converting binary integers to decimal, and then binary fractions to binary.
Binary integers to decimal: Algorithm
Step 1. To convert a binary integer to decimal, start by adding the left-most digit to 0.
Step 2. Next, multiply this by 2, and add the next digit in your number (as you progress from left to right) to this product. (In other words, multiply the current product in each step by 2 and add the current digit).
Step 3. Continue this process until there are no more digits left.
For example, let us convert the binary integer 1001 to decimal. Multiply the total by 2 and add the current digit, starting from the left.
-v1dec.gif)
Hence, the binary to decimal conversion of 1001 is 9.
Binary fractions to decimal
Step 1. For converting a binary fraction to decimal, start by adding the right-most digit to 0.
Step 2. Next, divide this by 2, add the next digit to this total (while progressing from right to left), and so on.
Step 3. Keep doing this until there are no more digits left.
For example, let's convert the fraction 0.1101 to decimal. Divide the total by 2 and add the current digit, starting from the right.
-o7u6l.gif)
As seen, the decimal representation of 0.1101 is 0.8125.
The math behind decimal-binary conversion
Any number in a number system can be represented as its base expansion, where 'base' is the number base - eg., base-2 being binary, base-10 being decimal, base-16 being hexadecimal, and so on.
So, any integer can be represented as follows:
-e6zer.gif)
where,
A is an integer
x is the digit value
b is the base value
For example, the integer 694 can be represented in base-10 and base-2 as:
-9iajx.gif)
Similarly, a fraction can be as follows:
-5jboh.gif)
where,
A is a fraction
x is the digit value
b is the base value
For example, the fraction 0. can be represented in base-10 and base-2 as:
-8fj99.gif)
Binary representation of non-terminating and recurring numbers
Some fractions that can be represented in the decimal system run into an infinite stream of 0's and 1's. These are the fractions that when converted to decimals in the decimal system, return an infinitely-long stream of digits after the period. Hence, they're called non-terminating and recurring numbers.
For example, consider the fraction 1/6. The decimal value of 1/6 is:
-uabk5.gif)
We see that the digit '6' keeps recurring after the period, unto infinity.
This is because, to finitely represent any given number in a base-n system, the denominator of that number must be a power of the base (b). In the above example, 6 is not a power of 10, so 1/6 can't be represented in the base-10 system in decimal format.
Similarly, those numbers with a denominator that are not a power of 2 can't be represented finitely in the base-2 system.
For example, consider 0.3. 0.3 = 3/10. Since 10 is not a power of 2, 0.3 can't be represented in the base-2 system.
-0moh5.gif)
We see that the 4 digits '1001' keep repeating after the decimal point to infinity. Hence, we can represent it as:
-9fl2n.gif)
Decimal to binary table
Here's a handy decimal-binary conversion table:

Programs for converting decimal to binary
Here's an example of programs for converting decimal numbers to binary numbers. The logic used is mostly the same for both - Java and Python.
Decimal to binary in Java
// How to convert a decimal number to binary
// Java program
import java.io.*;
class decimal-to-binary {
static void decimaltobinary(int x)
{
int[] binary = new int[32];
int i = 0;
while (x > 0) {
binary[i] = x % 2;
x = x / 2;
i++;
}
for (int j = i - 1; j >= 0; j--)
System.out.print(binary[j]);
}
// driver
public static void main(String[] args)
{
int x = 17;
decimaltobinary(x);
}
}
Decimal to binary in JavaScript
<script>
// How to convert a decimal number to binary
// Javascript program
// function for conversion
function decimaltobinary(x)
{
let binary = new Array(32);
let i = 0;
while (x > 0) {
binary[i] = x % 2;
x = Math.floor(x / 2);
i++;
}
for (let j = i - 1; j >= 0; j--)
document.write(binary[j]);
}
// driver
let n = 17;
decimaltobinary(x);
</script>
Decimal to binary in Python
# How to convert a decimal number to binary
# Python program
# function for conversion
def decimaltobinary(x):
binary = [0] * x;
i = 0;
while (x > 0):
binary[i] = x % 2;
x = int(x / 2);
i += 1;
for j in range(i - 1, -1, -1):
print(binary[j], end = "");
# driver
x = 17;
decimaltobinary(x);